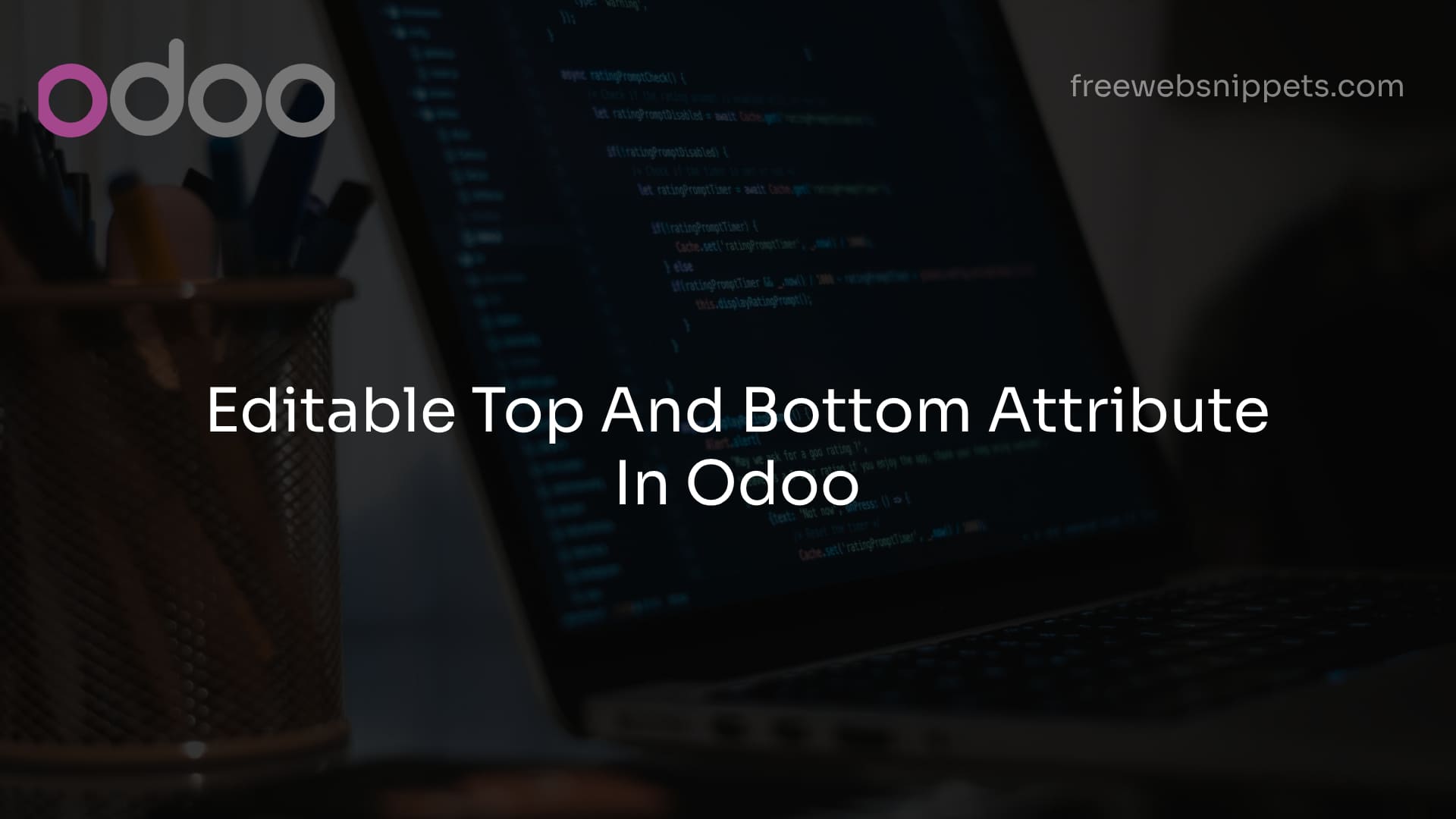
Learn how to effectively use archive and unarchive options in Odoo. This guide covers the steps to manage data visibility and keep your Odoo records organized.
Odoo, an all-in-one business management software, offers a wide range of functionalities to help businesses streamline their operations. Among these functionalities is the ability to archive and unarchive records. This feature is particularly useful for managing data that is no longer in active use but needs to be retained for future reference. Archiving allows users to hide records from everyday views without permanently deleting them. In this blog, we will walk you through the steps to implement and use the archive and unarchive options in Odoo.
1. Understanding the Archive/Unarchive Mechanism
2. Adding Archive/Unarchive Functionality to a Model
3. Adding Archive/Unarchive Actions to the User Interface
4. Testing the Archive/Unarchive Functionality
5. Enhancing the Archive/Unarchive Functionality
6. Conclusion
Archiving is a built-in feature in Odoo that enables users to temporarily remove records from active views. This is different from deleting records, as archived records can be easily restored (unarchived) when needed. Archiving is useful for managing records like old sales orders, inactive products, or outdated customer information, helping to keep your interface clean and focused on active data.
Data Retention: Archiving allows you to retain important data for historical reference without cluttering your active records.
Reversibility: Unlike deletion, archiving is reversible. You can easily unarchive records if they become relevant again.
Performance: Archiving reduces the amount of data displayed in views, potentially improving system performance.
In Odoo, the archive/unarchive mechanism is controlled by a boolean field named active. This field is typically included in models that support archiving. When the active field is set to False, the record is considered archived and is hidden from the default views. When it is set to True, the record is active and visible in the views.
'active' Field: A boolean field that determines whether a record is active ('True') or archived ('False').
Archive/Unarchive Actions: These actions toggle the value of the 'active' field.
To add archiving functionality to a custom model, you need to include the active field in the model definition. Most Odoo models already have this field, but if you're working with a custom model, here’s how to do it:
Add the 'active' field to your model's Python file:
from odoo import models, fields
class MyModel(models.Model):
_name = 'my.model'
_description = 'My Model'
name = fields.Char(string='Name', required=True)
description = fields.Text(string='Description')
active = fields.Boolean(string='Active', default=True)
Explanation:
'active' Field: The active field is defined as a boolean field with a default value of True. This means that records are active by default when they are created.
Odoo provides the archive/unarchive actions out of the box for models with the active field. However, if you want to customize the behavior or add additional logic, you can override these actions in your model’s Python file.
Here’s how to override the 'archive' method:
from odoo import models
class MyModel(models.Model):
_name = 'my.model'
def archive_records(self):
for record in self:
record.write({'active': False})
Explanation:
'archive_records' Method: This method sets the active field to False for each selected record, effectively archiving it.
Similarly, you can create an 'unarchive_records' method:
class MyModel(models.Model):
_name = 'my.model'
def unarchive_records(self):
for record in self:
record.write({'active': True})
Explanation:
'unarchive_records' Method: This method sets the active field to True, unarchiving the records.
To allow users to archive and unarchive records from the user interface, you need to add the appropriate actions to the list view.
In your module’s XML file, you can define the actions like this:
<record id="action_archive_my_model" model="ir.actions.server">
<field name="name">Archive</field>
<field name="model_id" ref="model_my_model"/>
<field name="binding_model_id" ref="model_my_model"/>
<field name="state">code</field>
<field name="code">
action = records.archive_records()
</field>
</record>
<record id="action_unarchive_my_model" model="ir.actions.server">
<field name="name">Unarchive</field>
<field name="model_id" ref="model_my_model"/>
<field name="binding_model_id" ref="model_my_model"/>
<field name="state">code</field>
<field name="code">
action = records.unarchive_records()
</field>
</record>
Explanation:
'action_archive_my_model': This server action calls the archive_records method when triggered.
'action_unarchive_my_model':span> This server action calls the unarchive_records method when triggered.
Next, you’ll want to add these actions to the list view of your model. This allows users to select records and apply the archive or unarchive action directly from the list view.
<record id="view_list_my_model" model="ir.ui.view">
<field name="name">my.model.list</field>
<field name="model">my.model</field>
<field name="arch" type="xml">
<tree>
<field name="name"/>
<field name="description"/>
</tree>
<form>
<header>
<button name="action_archive_my_model" type="action" string="Archive" class="oe_highlight"/>
<button name="action_unarchive_my_model" type="action" string="Unarchive"/>
</header>
</form>
</field>
</record>
Explanation:
List View Actions: The 'Archive' and 'Unarchive' buttons are added to the header of the form view. Users can select records and apply these actions directly from the list view.
After implementing the archive/unarchive functionality, it’s essential to test it to ensure it works as expected.
Install or upgrade your module in Odoo to load the new features.
- Navigate to the List View: Open the list view of the model where you added the archive/unarchive functionality.
- Select Records: Select one or more records from the list.
- Click Archive: Use the 'Archive' button to archive the selected records.
- Verify Archiving: After archiving, the records should disappear from the list view (if the list view only shows active records).
- Switch to Archived View: Use the search filter to show archived records (those with active set to False).
- Select Archived Records: Select one or more archived records.
- Click Unarchive: Use the 'Unarchive' button to restore the selected records.
- Verify Unarchiving: The records should reappear in the active list view.
- Missing Buttons: If the archive/unarchive buttons don’t appear, check the XML file to ensure the actions are correctly defined.
- Non-responsive Actions: If the buttons don’t work, verify that the server actions are correctly linked to the methods in the model.
Explanation:
Testing: Ensures that the archive/unarchive functionality works as expected and that users can easily manage active and archived records.
Debugging: Helps identify and resolve issues with the implementation.
Odoo allows for further customization of the archive/unarchive feature. Here are some ways to enhance it:
You can implement conditional logic to restrict when records can be archived. For example, you might want to prevent archiving records that are linked to active orders.
def archive_records(self):
for record in self:
if record.is_linked_to_active_order():
raise UserError('Cannot archive records linked to active orders.')
record.write({'active': False})
Explanation:
Conditional Archiving: Adds an extra layer of validation to ensure that only records meeting specific criteria can be archived.
You can notify users when records are archived or unarchived. This is particularly useful in workflows where archiving a record has downstream effects.
def archive_records(self):
for record in self:
record.write({'active': False})
# Send notification
self.env.user.notify_info(f'Record {record.name} has been archived.')
Explanation:
Notifications: Improve user awareness by sending notifications when important actions like archiving or unarchiving are performed.
For certain scenarios, you might want to automate the archiving process. For instance, you can create a scheduled action that automatically archives records older than a certain date.
def auto_archive_old_records(self):
old_records = self.search([('create_date', '<', fields.Date.today() - timedelta(days=365))])
old_records.write({'active': False})
Explanation:
Automated Archiving: Helps keep your database clean by automatically archiving outdated records based on specific criteria.
The archive and unarchive options in Odoo provide a flexible way to manage records that are no longer actively used but still need to be retained. By implementing these features, you can keep your user interface clean and focused, improve performance, and ensure that important data is preserved for future reference. Whether you’re working with standard Odoo models or custom models, adding archive/unarchive functionality is straightforward and highly beneficial.
With the steps outlined in this blog, you should now be equipped to implement and customize the archive/unarchive functionality in your Odoo applications. This not only enhances the user experience but also contributes to better data management practices.
Your email address will not be published. Required fields are marked *