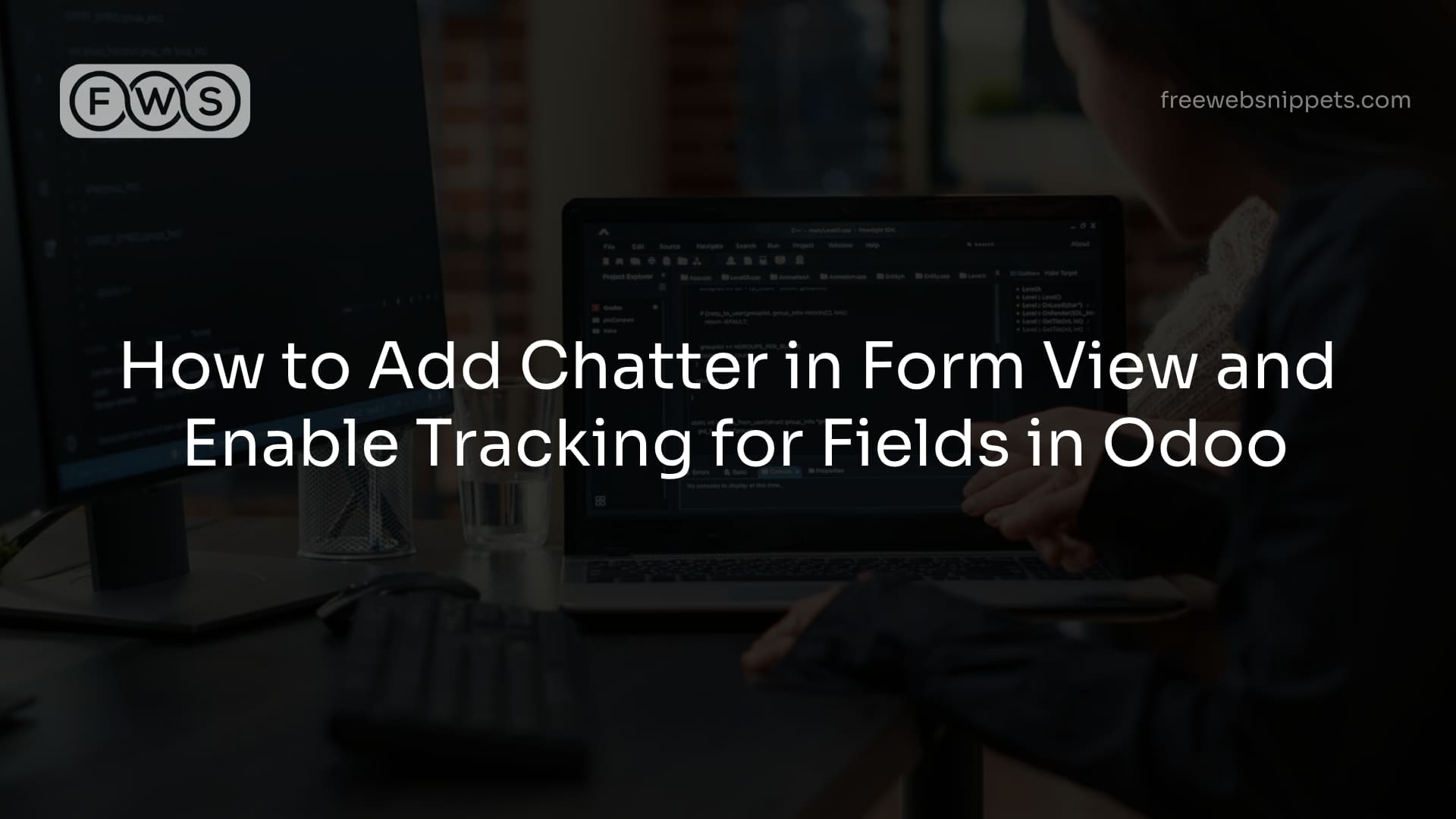
Discover how to add a Many2many field in Odoo to create complex data relationships. Follow this tutorial for easy integration and customization in your Odoo modules.
Odoo’s framework is known for its flexibility and powerful data management capabilities. One of the core features that makes this possible is the ability to establish relationships between different models. Among these relationships, the Many2many field is particularly useful for scenarios where records in one model can be linked to multiple records in another model and vice versa. This blog post will guide you step-by-step through the process of adding a Many2many field in Odoo, covering everything from model definitions to view customization.
Before we dive into the implementation, it’s essential to understand what a Many2many field is and why you might want to use it.
A Many2many field in Odoo represents a bidirectional relationship between two models where each record in one model can be linked to multiple records in another model, and each record in the second model can also be linked to multiple records in the first model. For example, consider a scenario where products can be linked to multiple categories, and each category can include multiple products. This relationship is effectively managed using a Many2many field.
Many2many fields are particularly useful when there’s a need to manage complex relationships between models. They provide flexibility and efficiency in managing data, ensuring that related records are easily accessible and modifiable. This can be useful in various business scenarios, such as tagging, categorizing, or managing membership in groups.
The first step in adding a Many2many field in Odoo is to define it in the corresponding models. This involves modifying the Python model files where the field will be used.
Let’s consider an example where we want to link products to multiple categories. We’ll add a Many2many field to the product.product model to link it to the product.category model.
First, locate the model file where you want to add the Many2many field. Typically, this file will be in the 'models' directory of your custom Odoo module.
Here is how you can define a Many2many field in the 'product.product' model:
from odoo import models, fields
class Product(models.Model):
_name = 'product.product'
_description = 'Product'
name = fields.Char(string='Product Name', required=True)
category_ids = fields.Many2many('product.category', string='Categories')
Explanation:
'category_ids': This is the name of the Many2many field. It’s common to name Many2many fields with an '_ids' suffix.
'product.category': This is the name of the related model that the Many2many field will reference. In this case, it refers to the product.category model.
'string='Categories': This defines the label that will be shown in the UI for this field.
With this code, the 'category_ids' field will allow you to associate multiple categories with a single product.
Next, you need to define the corresponding Many2many field in the 'product.category' model:
from odoo import models, fields
class ProductCategory(models.Model):
_name = 'product.category'
_description = 'Product Category'
name = fields.Char(string='Category Name', required=True)
product_ids = fields.Many2many('product.product', string='Products')
Explanation:
'product_ids': This Many2many field creates the reverse link from the 'product.category' model to the 'product.product' model.
By defining this field, each category can now be associated with multiple products, and the relationship will be maintained in both models.
Once you’ve defined the Many2many field in the models, the next step is to update the view to include this field. This involves modifying the XML file that defines the form or tree views for the model.
Let’s update the product form view to include the 'category_ids' Many2many field.
Here’s how you can do it:
<record id="view_product_form" model="ir.ui.view">
<field name="name">product.form</field>
<field name="model">product.product</field>
<field name="arch" type="xml">
<form string="Product">
<sheet>
<group>
<field name="name"/>
<field name="category_ids" widget="many2many_tags"/>
</group>
</sheet>
</form>
</field>
</record>
Explanation:
'
With this XML code, the 'category_ids' field will now appear in the product form view, enabling users to associate multiple categories with a product.
In addition to the form view, you might also want to display the Many2many field in a tree view (list view) for better data visualization.
Here’s how you can include the 'category_ids' field in the product tree view:
<record id="view_product_tree" model="ir.ui.view">
<field name="name">product.tree</field>
<field name="model">product.product</field>
<field name="arch" type="xml">
<tree string="Products">
<field name="name"/>
<field name="category_ids" widget="many2many_tags"/>
</tree>
</field>
</record>
Explanation:
'
This view allows users to quickly see which categories are associated with each product without having to open the form view.
After defining the Many2many field and updating the views, the next step is to test your implementation to ensure everything works as expected.
To apply your changes, restart the Odoo server. Once restarted, navigate to the relevant menu in the Odoo interface to check if the Many2many field is functioning correctly.
Create a few records in the 'product.product' model, then add category records using the Many2many field in the product form view. You should now be able to link multiple categories to a single product and vice versa.
Ensure that the categories are correctly linked to the products and that the relationship is reflected in both the product and category models.
To provide a better user experience, you can enhance the Many2many field with additional features, such as adding constraints, using context to prefill fields, and customizing the display of related records.
You can add constraints to the Many2many field to ensure data integrity. For example, you might want to ensure that a product is not assigned to the same category multiple times.
from odoo.exceptions import ValidationError
class Product(models.Model):
_name = 'product.product'
_description = 'Product'
name = fields.Char(string='Product Name', required=True)
category_ids = fields.Many2many('product.category', string='Categories')
@api.constrains('category_ids')
def _check_category_unique(self):
for record in self:
if len(record.category_ids) != len(set(record.category_ids.ids)):
raise ValidationError("A product cannot be assigned to the same category multiple times.")
Explanation:
'@api.constrains('category_ids')': This decorator is used to enforce a constraint on the 'category_ids' field.
'_check_category_unique': This method checks if the same category is assigned multiple times to the same product. If so, a 'ValidationError' is raised.
This constraint helps maintain the uniqueness of category assignments for each product.
You can use the 'context' attribute to prefill certain fields when creating new records through the Many2many field. For example, if you want to prefill a field in the 'product.category' model when adding a new category, you can do so as follows:
class Product(models.Model):
_name = 'product.product'
_description = 'Product'
name = fields.Char(string='Product Name', required=True)
category_ids = fields.Many2many('product.category', string='Categories', context="{'default_name': 'New Category'}")
Explanation:
'context="{'default_name': 'New Category'}"': This line pre-fills the 'name' field in the 'product.category' model with the default value 'New Category' when a new category is added.
This feature can streamline data entry and reduce the chances of errors.
You can customize how the related records are displayed in the Many2many field by using the 'tree' and 'form' views. This allows you to control how the related categories appear when selected in the product form view.
<field name="category_ids">
<tree string="Categories">
<field name="name"/>
</tree>
</field>
'
This customization helps ensure that the related records are presented clearly and concisely in the UI.
Adding a Many2many field in Odoo is a powerful way to manage complex relationships between models. By following the steps outlined in this blog, you can successfully implement a Many2many field in your custom Odoo modules, allowing users to associate records across different models seamlessly.
Whether you’re managing product categories, user groups, or other related records, the Many2many field provides the flexibility and efficiency needed to handle complex data relationships in Odoo.
As you continue to develop in Odoo, you’ll find that Many2many fields are just one of many powerful tools at your disposal. With practice and experience, you’ll be able to leverage these fields to create even more sophisticated and dynamic applications.
This blog post provides a comprehensive guide to adding a Many2many field in Odoo, covering all essential steps while ensuring the content remains relevant and informative for developers at all levels.
Your email address will not be published. Required fields are marked *