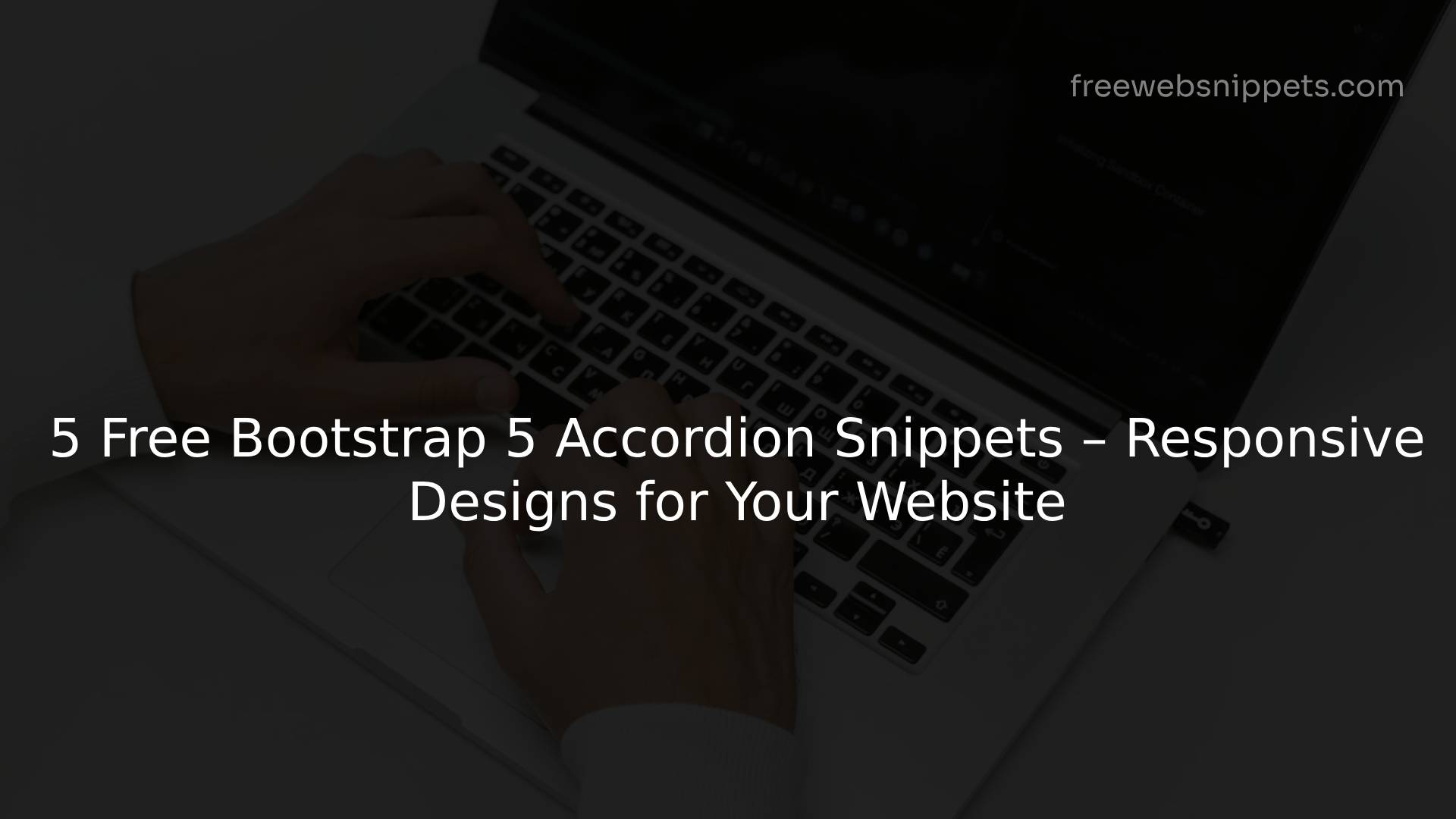
Explore how to define models in Odoo with this comprehensive guide. Learn the basics of Odoo models, their structure, and how they interact with other components to build powerful applications
Odoo, a popular open-source ERP platform, is known for its flexibility and extensibility. One of the core components that make this possible is its powerful ORM (Object-Relational Mapping) system, which allows developers to define models that map directly to database tables. These models are the backbone of Odoo, handling data storage, business logic, and more.
In this blog, we will delve into the process of defining models in Odoo, explaining each step with detailed examples to give you a thorough understanding. By the end of this guide, you’ll have the knowledge to create your own models in Odoo and customize the platform to meet your specific business needs.
1. Understanding the Concept of Models in Odoo
2. Setting Up Your Development Environment
3. Creating the Basic Structure of a Model
4. Exploring Field Types in Odoo Models
5. Adding Business Logic to Models
6. Defining Views and Actions for Your Model
7. Testing and Debugging Your Model
Before we dive into the technical details, it’s important to understand what models are and their role in Odoo.
What is a Model? In Odoo, a model is a Python class that defines the structure of a database table. It encapsulates the business logic, including how data is created, updated, and deleted. Each model typically corresponds to a single table in the database, with its fields representing columns in that table.
Models in Odoo are designed to be highly extensible. This means you can not only create new models but also inherit and extend existing ones to add new features or modify behavior. This flexibility is one of the key strengths of Odoo’s framework.
- Fields: Represent the data attributes (columns) of the model (table).
- Methods: Define business logic and operations that can be performed on the data.
- Constraints: Ensure data integrity by enforcing rules at the database level.
- Relations: Define relationships between different models (tables).
To define models in Odoo, you need to have a proper development environment set up. Here’s a quick overview:
1. Install Odoo: Make sure Odoo is installed on your system. Odoo 17 is the latest version at the time of writing, and this guide will be based on it. If you need help with installation, refer to the official Odoo documentation or my previous guide.
2. Create a Custom Module: Before defining models, you’ll typically create a custom module to house them. A module is a self-contained package that can include models, views, controllers, and other components.
3. Set Up Python: Ensure you have Python 3.8+ installed, as Odoo models are written in Python. Using a virtual environment is recommended to manage dependencies.
4. Editor and Tools: Use a code editor like Visual Studio Code or PyCharm, which provides support for Python and Odoo development.
Now that your environment is ready, let’s create a basic model in Odoo. We’ll walk through the process step by step.
1. Create the Model File: In your custom module directory, create a models folder if it doesn’t already exist. Inside this folder, create a Python file for your model. For example: 'custom_module/models/custom_model.py'
2. Define the Model Class: Open the custom_model.py file in your code editor. Start by importing the necessary modules and defining your model class:
from odoo import models, fields
class CustomModel(models.Model):
_name = 'custom.model' # The unique name of your model
_description = 'Custom Model Description' # A brief description of your model
name = fields.Char(string='Name', required=True) # A character field
description = fields.Text(string='Description') # A text field
active = fields.Boolean(string='Active', default=True) # A boolean field
In this example:
_name: This is the internal name of your model. It must be unique within the Odoo instance.
_description: This is a human-readable description of the model.
Fields: We’ve defined three fields—name, description, and active. Each field is an instance of a specific field type, such as Char, Text, or Boolean.
3. Add the Model to the __init__.py File: To ensure that Odoo recognizes your model, you need to import it in the '__init__.py' file located in the 'models' directory.
from . import custom_model
4. Define the Model in the Manifest File: Finally, make sure your model is included in the module’s manifest file (__manifest__.py). This file tells Odoo what components your module contains.
{
'name': 'Custom Module',
'version': '1.0',
'author': 'Your Name',
'depends': ['base'],
'data': [
'views/custom_model_views.xml', # We’ll define views later
],
'installable': True,
'application': True,
}
Fields are the building blocks of models in Odoo. They define the data structure by specifying the types of data the model will store. Odoo provides a wide variety of field types to accommodate different kinds of data.
Here’s an overview of some commonly used field types:
1. Basic Fields:
- Char: Stores a string of text, typically used for names, titles, or identifiers.
- Text: Used for longer pieces of text, such as descriptions or comments.
- Integer: Stores whole numbers.
- Float: Stores decimal numbers, useful for monetary values or percentages.
- Boolean: Stores True or False values, commonly used for flags or toggles.
- Date: Stores a date value.
- Datetime: Stores both date and time.
name = fields.Char(string='Name', required=True)
price = fields.Float(string='Price')
is_active = fields.Boolean(string='Is Active', default=True)
start_date = fields.Date(string='Start Date')
timestamp = fields.Datetime(string='Timestamp')
2. Relational Fields: Relational fields define relationships between models, allowing you to create links between different tables.
- Many2one: Creates a many-to-one relationship, where many records in the current model can be linked to a single record in another model.
category_id = fields.Many2one('product.category', string='Category')
- One2many: Creates a one-to-many relationship, where a single record in the current model can be linked to many records in another model.
line_ids = fields.One2many('custom.line', 'order_id', string='Lines')
- Many2many: Creates a many-to-many relationship, where many records in the current model can be linked to many records in another model.
tag_ids = fields.Many2many('custom.tag', string='Tags')
3. Computed Fields: Computed fields are fields whose values are computed based on other fields. These fields are not stored in the database but are calculated on the fly when accessed.
total_price = fields.Float(string='Total Price', compute='_compute_total_price')
@api.depends('price', 'quantity')
def _compute_total_price(self):
for record in self:
record.total_price = record.price * record.quantity
4. Selection Fields: Selection fields allow you to define a fixed list of choices for a field. The user can select one option from the list.
status = fields.Selection([
('draft', 'Draft'),
('confirmed', 'Confirmed'),
('done', 'Done')
], string='Status', default='draft')
Business logic in Odoo is implemented using methods within your model classes. These methods can be triggered by user actions, automatically based on certain conditions, or called from other parts of the system.
1. Standard Methods:
- create(): This method is called when a new record is created.
- write(): This method is called when an existing record is updated.
- unlink(): This method is called when a record is deleted.
You can override these methods to add custom behavior:
def create(self, vals):
# Custom logic before creating a record
record = super(CustomModel, self).create(vals)
# Custom logic after creating a record
return record
def write(self, vals):
# Custom logic before updating a record
result = super(CustomModel, self).write(vals)
# Custom logic after updating a record
return result
def unlink(self):
# Custom logic before deleting a record
result = super(CustomModel, self).unlink()
# Custom logic after deleting a record
return result
2. Onchange Methods: Onchange methods are triggered when a field value changes. These methods can be used to dynamically update other fields based on user input.
@api.onchange('price')
def _onchange_price(self):
if self.price < 0:
self.price = 0 # Ensure the price is not negative
return {
'warning': {
'title': "Incorrect 'price' value",
'message': "The price cannot be negative.",
}
}
3. Constrains: Constraints are used to enforce business rules at the database level. For example, you can use constraints to ensure that a field is unique or that a value meets certain conditions.
@api.constrains('price')
def _check_price(self):
if self.price < 0:
raise ValidationError("The price cannot be negative.")
Once your model is defined, you’ll need to create views and actions to interact with it through the Odoo interface.
1. Create a View: Views define how your model data is presented to the user. Odoo supports several types of views, including form views, tree views, kanban views, and more.
Example of a simple form view:
<record id="view_custom_model_form" model="ir.ui.view">
<field name="name">custom.model.form</field>
<field name="model">custom.model</field>
<field name="arch" type="xml">
<form string="Custom Model">
<sheet>
<group>
<field name="name"/>
<field name="description"/>
<field name="active"/>
</group>
</sheet>
</form>
</field>
</record>
2. Define an Action: Actions define how the model is accessed and what happens when a user interacts with it. For example, you can define a menu item that opens a specific view of your model.
<record id="action_custom_model" model="ir.actions.act_window">
<field name="name">Custom Models</field>
<field name="res_model">custom.model</field>
<field name="view_mode">tree,form</field>
</record>
<menuitem id="menu_custom_model" name="Custom Models"
action="action_custom_model" parent="base.menu_custom"/>
3. Security and Access Control: Finally, define access rights for your model to ensure that only authorized users can view or modify the data.
<record id="model_custom_model_access_user" model="ir.model.access">
<field name="name">custom.model access</field>
<field name="model_id" ref="model_custom_model"/>
<field name="group_id" ref="base.group_user"/>
<field name="perm_read" eval="1"/>
<field name="perm_write" eval="1"/>
<field name="perm_create" eval="1"/>
<field name="perm_unlink" eval="1"/>
</record>
After defining your model, it’s crucial to test it thoroughly to ensure everything works as expected.
1. Load the Module: Install your custom module in Odoo by updating the app list and installing it from the Apps menu.
2. Test Basic Operations: Create, update, and delete records in your model to ensure that all fields, methods, and constraints are functioning correctly.
3. Check for Errors: Use Odoo’s logging and debugging tools to catch any errors or issues. The Odoo log file (odoo.log) is an excellent resource for troubleshooting.
4. Use Odoo’s Test Framework: Odoo provides a built-in testing framework that allows you to write automated tests for your models. Consider creating test cases to validate your model’s behavior under different scenarios.
Defining models in Odoo is a fundamental skill for any developer working with the platform. Models form the core of Odoo’s data structure, and understanding how to create and extend them allows you to build robust, customized solutions that meet your business needs.
In this guide, we’ve covered the basics of creating models in Odoo, including defining fields, adding business logic, creating views, and testing your models. With this knowledge, you can confidently start building your own models in Odoo, tailoring the platform to fit your requirements.
As you continue to explore Odoo development, you’ll find that models are just one part of the larger ecosystem. Combining models with views, controllers, and other components allows you to create comprehensive applications that leverage the full power of Odoo.
Happy coding, and may your Odoo models always be clean, efficient, and powerful!
Your email address will not be published. Required fields are marked *