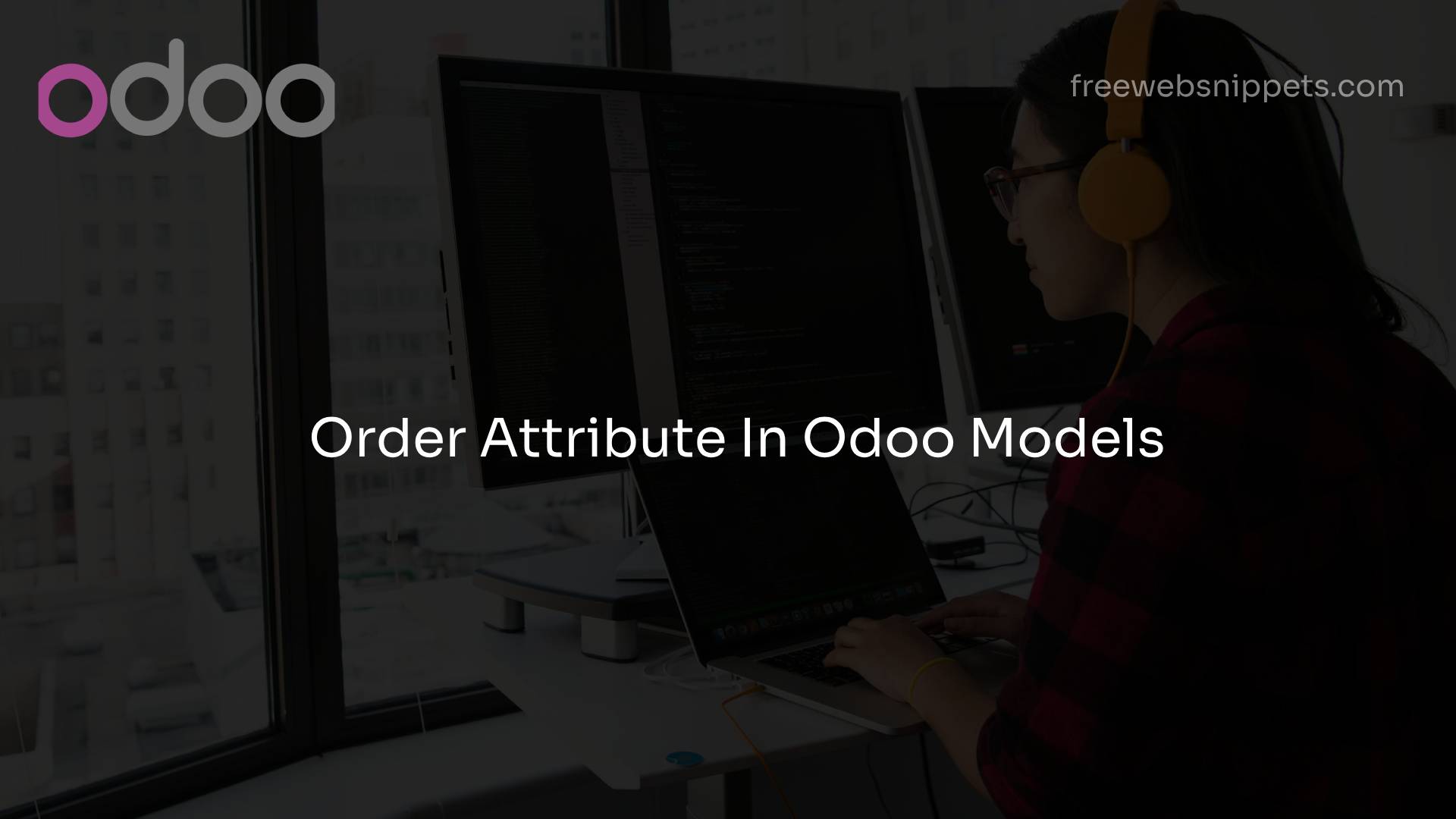
Learn how to show alerts in Odoo form view to enhance user experience. Implement notifications, warnings, and custom messages easily.
Displaying alerts in Odoo's form view can enhance user experience by providing important notifications or warnings directly within the interface. Alerts can be used for various purposes, such as indicating validation issues, confirmations, or general information. In this blog, we'll explore how to implement alerts in Odoo's form view effectively.
In Odoo, alerts can be implemented using the notifications feature or by creating custom messages in the form view. This allows you to inform users of important updates or actions they need to take. Alerts can be temporary or persistent, depending on the context and requirement.
To show alerts in a form view, you can leverage the Odoo warning notification system. Here’s how to do it:
from odoo import models, api, _
class CustomModel(models.Model):
_name = 'custom.model'
@api.model
def create(self, vals):
record = super(CustomModel, self).create(vals)
# Show alert after creating a record
record.message_post(body=_("Record created successfully!"), message_type='notification')
return record
In this example:
Odoo also provides a way to show warning messages, which can be useful for alerting users about potential issues. You can use the warn method to display a warning when certain conditions are met.
from odoo.exceptions import UserError
@api.constrains('some_field')
def _check_some_field(self):
for record in self:
if record.some_field < 0:
raise UserError(_("The value cannot be negative!"))
In this code snippet:
If you want to create a more customized alert within the form view, you can do so by using the alert class in your XML view definitions:
<form>
<sheet>
<div class="alert alert-warning">
<strong>Warning!</strong> Please check the data before saving.
</div>
</sheet>
</form>
In this example:
Showing alerts in Odoo form views is essential for improving user experience and ensuring data integrity. By utilizing the notification system, warning messages, and custom alerts, you can effectively communicate important information to users in real-time. Whether you need to notify users of successful actions or warn them about potential issues, Odoo provides flexible options to implement alerts within your forms.
Your email address will not be published. Required fields are marked *