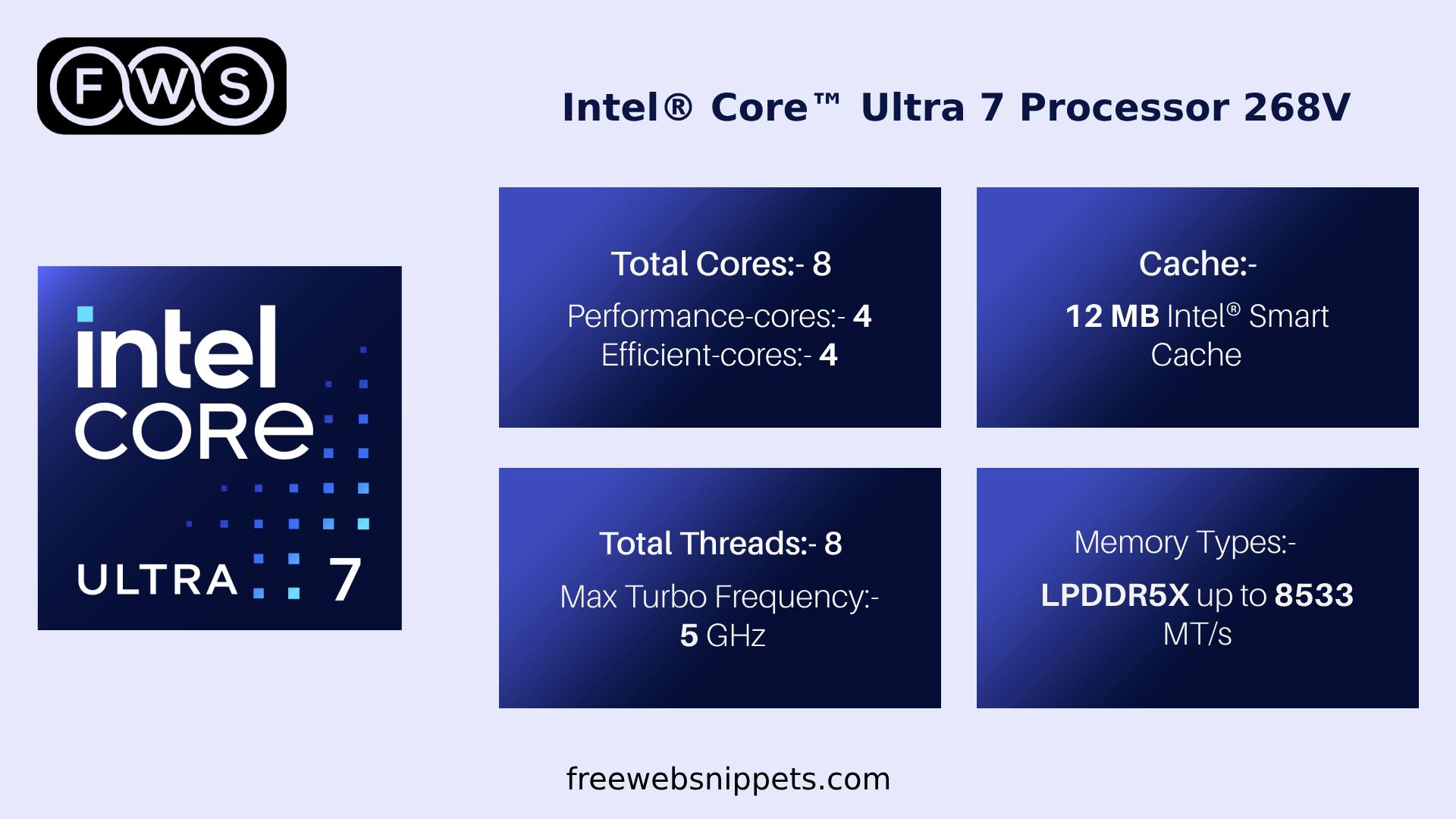
Learn how to prevent the closing of a wizard in Odoo with practical code examples and detailed explanations for effective implementation.
Wizards in Odoo are transient forms. They enable users to fulfill multi-step processes. Also to capture input efficiently. Wizards serve as a vital interface for actions. These actions mandatorily require user engagement. They could be data collection or action verification. Yet, certain situations might surface. There could be a need to stop users from closing a wizard. The condition? Users should meet a specific criteria. That criterion could be filling in necessary fields. This blog will instruct you on implementing such functionality in Odoo. The implementation is about not letting users close a wizard. Wizard should remain open until they satisfy a certain condition. That condition is filling in requested fields. The goal of this blog is to guide you through the process more efficiently.
Wizard is a transient model. It's an Odoo model. It facilitates user actions. Those actions need input or confirmation. They progress through a series of steps. Wizard is usually shown as a form. Users can input data in this form. On completion wizard can trigger specific actions. Wizard is designed to be temporary. It's used often to simplify complex workflows or tasks.
To stop users from closing wizard an override is necessary. It should stop this without completing the required fields. You can override the action_cancel method. Below there's an example. The example shows how to implement this functionality in a custom wizard model.
from odoo import models, api, fields, exceptions
class MyWizard(models.TransientModel):
_name = 'my.wizard'
_description = 'My Custom Wizard'
name = fields.Char(string='Name', required=True)
description = fields.Text(string='Description')
@api.model
def action_cancel(self):
# Check if required fields are filled
if not self.name or not self.description:
raise exceptions.UserError(
"You cannot close this wizard until you fill out the Name and Description fields."
)
# If conditions are met, proceed with the standard cancel behavior
return super(MyWizard, self).action_cancel()
@api.model
def action_confirm(self):
# Logic for confirming the wizard's actions
self.env['some.model'].create({
'name': self.name,
'description': self.description,
})
return {'type': 'ir.actions.act_window_close'}
Wizard is transient model named my.wizard. It has two fields. One is name a required character field. The other field is description, a text field.
This method is overridden. It implements custom behavior when user tries to cancel or close the wizard. It verifies if the required fields (name and description) are filled. If any of the required fields is empty, a UserError is raised. The message requests the user to fill in the necessary information. This way wizard stays open and user is guided to finish necessary actions.
This method manages the logic of confirming wizard's actions. When user affirms the wizard, a new record is born in different model (e.g., some.model). It uses data entered in wizard fields. If the record creation is successful the method provides an action to close wizard.
When preventing wizard closure keep certain best practices in mind. These enhance user experience:
To impede wizard's closure in Odoo is crucial. It ensures users finish necessary inputs before continuing. Overriding action_cancel method goes hand in hand with incorporating proper error handling. This guides users effectively throughout process. This technique boosts reliability of Odoo applications. It also betters overall user experience.
Clear instructions and feedback mechanisms are in place. Wizards become potent tools for data collection and workflow management.
Your email address will not be published. Required fields are marked *