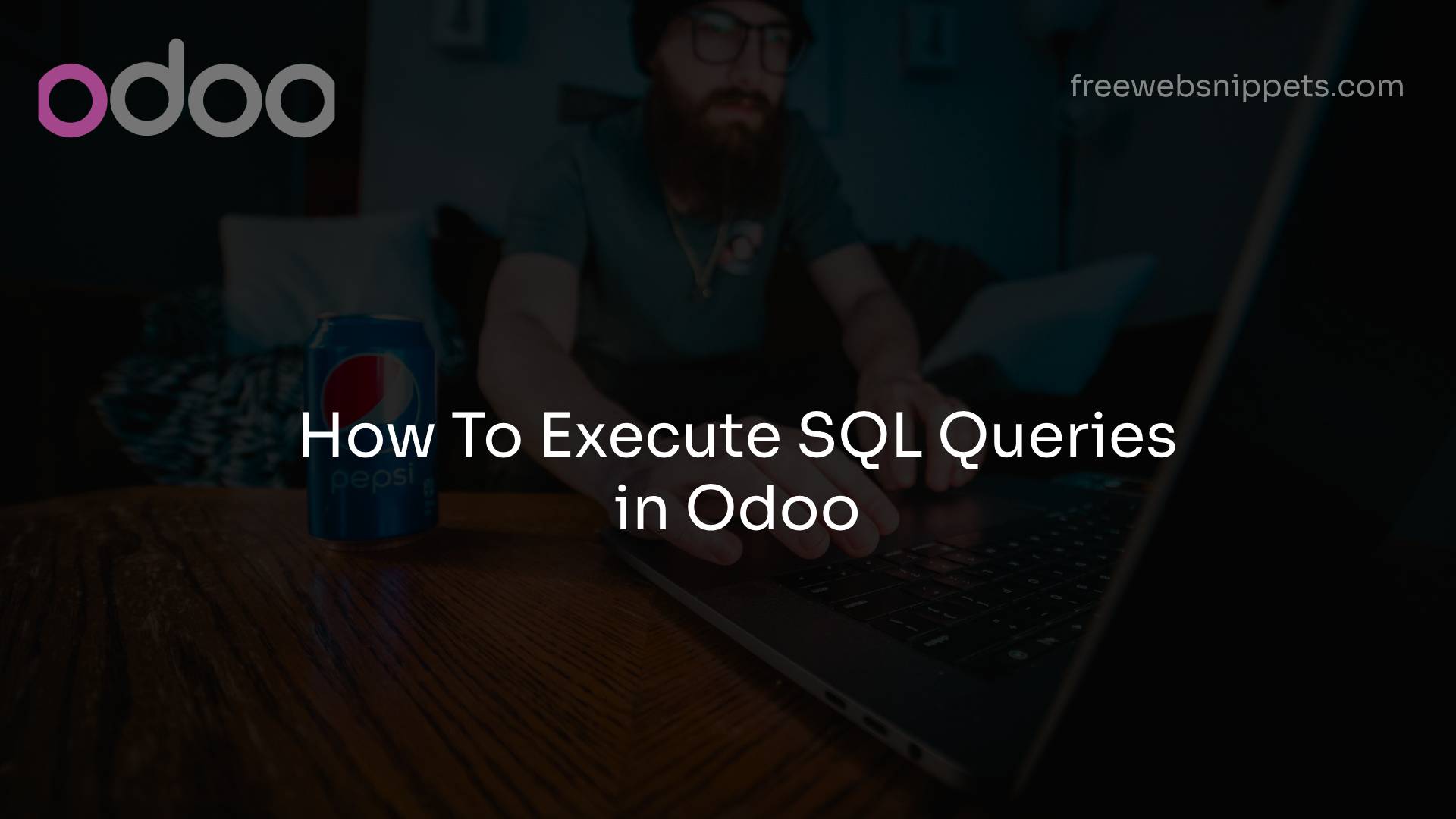
Learn how to use the default_get function in Odoo to set default values for fields when creating new records. Step-by-step guide included.
The Default Get function in Odoo is a powerful feature that allows developers to set default values for fields when creating new records. This function can enhance user experience by pre-filling fields based on specific logic, reducing the need for users to enter repetitive data manually. In this blog post, we will explore how to implement the default_get function in Odoo with practical examples.
The default_get function is a special method in Odoo that is automatically called when creating new records. It allows you to define default values for fields based on specific criteria or existing data. By overriding this method, you can customize the default values based on various conditions, such as user preferences, existing records, or other contextual data.
To use the default_get function, you need to inherit the model you want to customize. Here’s how to do it:
Let’s say we want to set default values for the res.partner model, which manages contacts in Odoo. You first need to create a new class that inherits from the res.partner model.
from odoo import models, fields
class CustomPartner(models.Model):
_inherit = 'res.partner'
Next, you can override the default_get
method to define default values for specific fields:
def default_get(self, fields):
res = super(CustomPartner, self).default_get(fields)
# Set default values
if 'customer' in fields:
res['customer'] = True # Set the default customer flag to True
if 'supplier' in fields:
res['supplier'] = False # Set the default supplier flag to False
return res
In this example:
default_get
method using super()
to ensure that we maintain any default values defined in the base model.fields
list. If they are, we set their default values accordingly.You can also use the context to set default values based on certain conditions. For example, if you want to set a default value for a field based on the current user’s preferences:
def default_get(self, fields):
res = super(CustomPartner, self).default_get(fields)
# Check the context for specific conditions
if self.env.context.get('default_customer_type') == 'individual':
res['customer'] = True
res['supplier'] = False
else:
res['customer'] = False
res['supplier'] = True
return res
In this example:
The default_get method can also be used to set default values for related fields. For instance, if you want to set a default country for new partners:
def default_get(self, fields):
res = super(CustomPartner, self).default_get(fields)
# Set default country for new partners
if 'country_id' in fields:
default_country = self.env['res.country'].search([('code', '=', 'US')], limit=1)
res['country_id'] = default_country.id if default_country else False
return res
In this case:
fields
list and search for the country with the code 'US'.When implementing the default_get method, consider the following best practices:
super()
.default_get
to maintain readability and performance.The default_get function in Odoo is a valuable tool for developers looking to enhance user experience by pre-filling fields with relevant data when creating new records. By following the steps outlined in this blog post, you can effectively implement and customize the default_get method to meet your specific business requirements.
For more Odoo development tips and tricks, be sure to check our website Free web snippets.
Your email address will not be published. Required fields are marked *