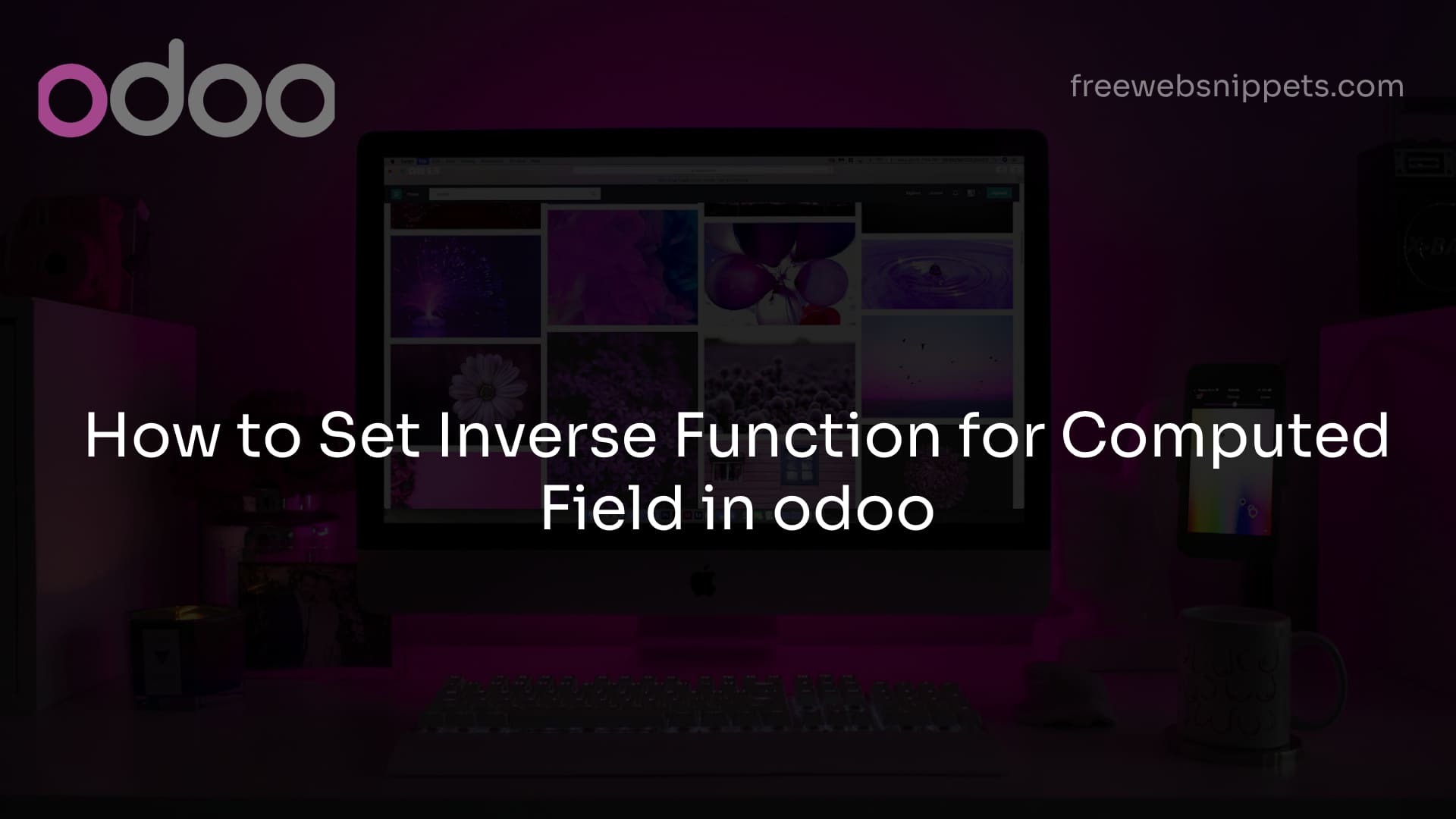
Learn how to use Python constraints in Odoo to ensure data integrity with @api.constraints. Implement custom validation logic in your Odoo models.
In Odoo, constraints are critical to maintaining data integrity by enforcing rules at both the database and application levels. While SQL constraints help restrict data at the database level, Python constraints allow developers to apply custom validation logic within Odoo’s business models. In Odoo, Python constraints are implemented using the @api.constraints decorator. In this blog, we’ll explore how to use Python constraints in Odoo, focusing on the @api.constraints decorator and provide practical examples of its application.
Python constraints are rules that you define in your Odoo model’s Python code. These constraints are validated during record creation and modification. Unlike SQL constraints, Python constraints allow for more complex and flexible validations. By using Python constraints, you can perform validation checks based on multiple fields or custom conditions that go beyond simple database rules.
In Odoo, you can define Python constraints using the @api.constraints decorator. This decorator is used with methods that check certain conditions and raise validation errors if the conditions are not met. These methods are automatically triggered when records are created or updated in the database.
Here’s an example of a Python constraint that ensures a product’s sale price cannot be negative:
from odoo import models, fields, api
from odoo.exceptions import ValidationError
class ProductTemplate(models.Model):
_inherit = 'product.template'
@api.constrains('list_price')
def _check_sale_price(self):
for record in self:
if record.list_price < 0:
raise ValidationError('The sale price cannot be negative.')
In this example, the method _check_sale_price checks if the list_price is less than zero for any product. If the condition is met, a ValidationError is raised, preventing the record from being saved.
Whenever a record is created or updated, Odoo will automatically call any methods decorated with @api.constraints. These methods will validate the record and, if necessary, raise an error to prevent invalid data from being saved. In the example above, the system prevents users from setting a negative price for a product, ensuring data integrity.
Python constraints can also validate multiple fields at once. For example, you might want to ensure that a product's cost price is not greater than its sale price. Here’s how you can implement such a constraint:
from odoo import models, fields, api
from odoo.exceptions import ValidationError
class ProductTemplate(models.Model):
_inherit = 'product.template'
@api.constrains('list_price', 'standard_price')
def _check_price(self):
for record in self:
if record.list_price < record.standard_price:
raise ValidationError('The sale price cannot be less than the cost price.')
In this case, the constraint checks if the list_price (sale price) is lower than the standard_price (cost price). If the sale price is lower than the cost price, a ValidationError will be raised, ensuring that the business logic is maintained.
Python constraints in Odoo give you powerful tools to enforce business rules and ensure data consistency at the application level. By using the @api.constraints decorator, you can implement complex validation logic that works across multiple fields and conditions. This ensures that your data stays accurate and consistent, preventing users from inadvertently saving incorrect data.
For more detailed information on Odoo menu customization, refer to the Free web snippets.
Your email address will not be published. Required fields are marked *