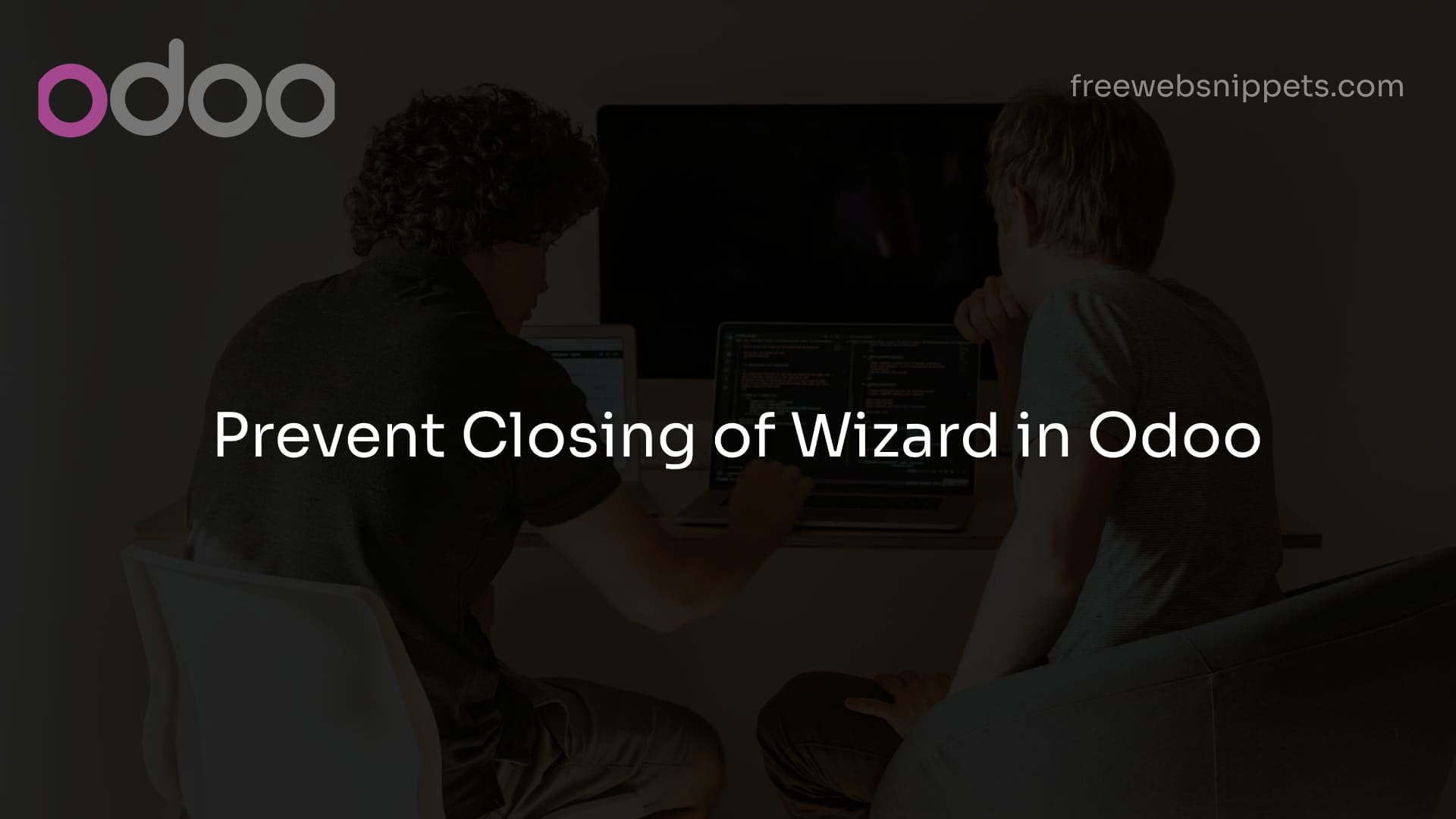
Learn how to add settings for your custom module in Odoo using res.config.settings. Enable flexibility and user-friendly configuration options.
In Odoo, adding settings for a module is done through the res.config.settings model. This model allows developers to define configuration options that can be managed through the Odoo user interface, providing flexibility to change certain behaviors of a module without altering the code. In this blog, we’ll go over how to add settings for your custom Odoo module using res.config.settings.
Using res.config.settings allows the administrator to configure module-specific settings from the backend, making it easy to adapt the module to different business needs. Instead of hardcoding values, administrators can set options that control how the module functions.
Below are the steps to create settings for a module in Odoo:
The first step is to create a Python class that inherits the res.config.settings model. This class will define the settings fields and their default values.
from odoo import models, fields
class MyModuleSettings(models.TransientModel):
_inherit = 'res.config.settings'
enable_feature = fields.Boolean("Enable Feature", default=False)
module_option = fields.Char("Module Option")
def set_values(self):
super(MyModuleSettings, self).set_values()
self.env['ir.config_parameter'].sudo().set_param('my_module.enable_feature', self.enable_feature)
self.env['ir.config_parameter'].sudo().set_param('my_module.module_option', self.module_option)
@api.model
def get_values(self):
res = super(MyModuleSettings, self).get_values()
res.update(
enable_feature=self.env['ir.config_parameter'].sudo().get_param('my_module.enable_feature'),
module_option=self.env['ir.config_parameter'].sudo().get_param('my_module.module_option')
)
return res
In this example, we are adding two fields: enable_feature (a boolean) and module_option (a character field). These fields will be available in the settings menu of our custom module.
Next, we need to create an XML view to display these settings in the Odoo interface. This is done by defining a form view for res.config.settings.
<record id="my_module_settings_view" model="ir.ui.view">
<field name="name">my.module.settings.view</field>
<field name="model">res.config.settings</field>
<field name="arch" type="xml">
<form string="My Module Settings">
<group>
<field name="enable_feature"/>
<field name="module_option"/>
</group>
</form>
</field>
</record>
This XML code defines a simple form view for the settings. The enable_feature and module_option fields are displayed in the settings form for the administrator to modify.
To make the settings accessible, you need to add a menu item in the Odoo backend. This is done by creating a new menu record.
<record id="menu_my_module_settings" model="ir.ui.menu">
<field name="name">Module Settings</field>
<field name="parent_id" ref="base.menu_administration"/>
<field name="action" ref="my_module_settings_action"/>
</record>
<record id="my_module_settings_action" model="ir.actions.act_window">
<field name="name">Module Settings</field>
<field name="res_model">res.config.settings</field>
<field name="view_mode">form</field>
<field name="target">inline</field>
</record>
This will add a "Module Settings" menu item under the "Administration" menu, making it easy for the administrator to navigate and change the settings for the module.
Using res.config.settings in Odoo to create module settings allows for a more dynamic and configurable module. With just a few steps, you can allow users to adjust specific behaviors of your module without changing the source code, making your module more user-friendly and adaptable.
Your email address will not be published. Required fields are marked *