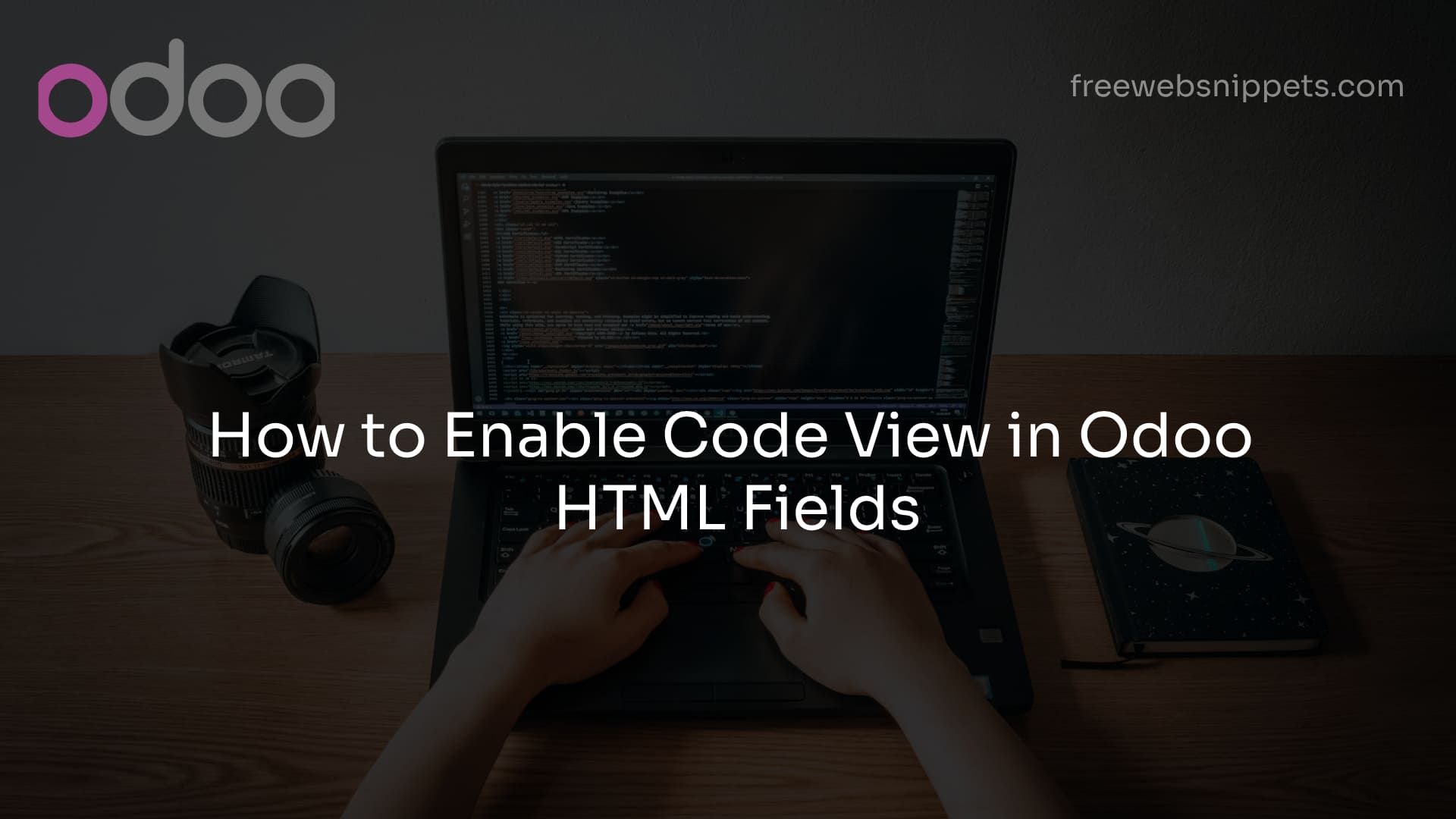
Master the techniques to apply domain filters for menus in Odoo and set default values using context. Enhance your Odoo customization skills with this comprehensive guide.
Odoo, a powerful open-source ERP platform, provides extensive customization options that allow developers to tailor the system to specific business needs. Two critical aspects of this customization are the ability to apply domains to menus and set default values using context. These features enhance the user experience by filtering records and pre-populating forms with default data. In this blog, we will walk through the steps to apply a domain for a menu in Odoo and set default values using context, focusing on practical examples.
1. Applying Domain for a Menu in Odoo
2. Setting Default Values Using Context in Odoo
3. Combining Domains and Context for Enhanced Functionality
4. Advanced Use Cases and Best Practices
5. Conclusion
Before we dive into the implementation, it’s essential to understand what domains and contexts are in Odoo:
Domains: A domain in Odoo is a condition or filter that is applied to records to limit the data displayed in views. Domains are used extensively to filter records in list views, search views, and menus.
Context: Context in Odoo provides additional information to actions, methods, and views. It is often used to set default values in forms, control visibility, or pass extra parameters.
Applying a domain to a menu allows you to control which records are displayed when a user navigates to that menu. This is particularly useful for creating custom menus that only show a specific subset of data.
In Odoo, menus are defined in XML files using the '
To apply a domain to a menu, follow these steps:
Locate the Action: Find or create the action associated with the menu. The action should point to the model you want to filter.
Add the Domain: Define the domain directly in the action using the 'domain' attribute.
Here’s an example where we create a menu that only displays active customers:
<record id="action_active_customers" model="ir.actions.act_window">
<field name="name">Active Customers</field>
<field name="res_model">res.partner</field>
<field name="view_mode">tree,form</field>
<field name="domain">[('customer_rank', '>', 0), ('active', '=', True)]</field>
</record>
Explanation:
'res_model': Specifies the model ('res.partner') for which the action is created.
'domain': Filters records where 'customer_rank' is greater than 0 and 'active' is 'True'. This ensures that only active customers are displayed.
Next, link the action to a menu item so that the domain is applied when users navigate through the menu.
<menuitem id="menu_active_customers" name="Active Customers" parent="base.menu_sales" action="action_active_customers"/>
Explanation:
'menu_active_customers': The unique ID for the menu item.
'action_active_customers': Links the menu to the action that has the domain.
After defining the menu and action, install or upgrade your module in Odoo. Navigate to the new menu and ensure that only the records matching the domain criteria are displayed.
Testing Steps:
'Navigate to the Menu': Go to the "Active Customers" menu.
'Verify the Filter': Ensure that only active customers are listed.
Setting default values using context is a powerful feature in Odoo that simplifies data entry by pre-populating forms with specific values. This can be particularly useful in scenarios where certain fields should have predefined values based on the user's action.
Context is a dictionary that can be passed to various Odoo methods and views. It provides additional information that can be used to set default values, filter records, or control behavior. When setting default values, the context is typically used in actions or views.
You can set default values in actions by adding a 'context' attribute. Here’s an example of an action that opens a form to create a new customer, with the 'country_id' field set to a default value:
<record id="action_create_customer_usa" model="ir.actions.act_window">
<field name="name">Create Customer USA</field>
<field name="res_model">res.partner</field>
<field name="view_mode">form</field>
<field name="context">{'default_country_id': 233}</field>
</record>
Explanation:
'default_country_id': Sets the default country to the United States (ID 233) when creating a new customer.
You can also set default values in specific views by adding a 'context' attribute to the 'form' tag. Here’s an example where the 'is_company' field is set to 'True' by default when creating a new partner record:
<record id="view_form_partner_company" model="ir.ui.view">
<field name="name">res.partner.form.company</field>
<field name="model">res.partner</field>
<field name="arch" type="xml">
<form string="Partner" context="{'default_is_company': True}">
<group>
<field name="name"/>
<field name="is_company"/>
<field name="company_type"/>
</group>
</form>
</field>
</record>
Explanation:
'default_is_company': Automatically sets the 'is_company' field to 'True' when creating a new record using this form view.
You can also pass context in server actions, which allows you to set default values when executing specific server-side operations. For example:
<record id="action_create_invoice_with_defaults" model="ir.actions.server">
<field name="name">Create Invoice with Defaults</field>
<field name="model_id" ref="account.model_account_move"/>
<field name="binding_model_id" ref="account.model_account_move"/>
<field name="state">code</field>
<field name="code">
action = env['account.move'].with_context(default_move_type='out_invoice', default_partner_id=1).create({})
</field>
</record>
Explanation:
'default_move_type': Sets the default move type to 'out_invoice' (customer invoice).
'default_partner_id': Pre-sets the customer to the one with ID '1'.
Once you've set the default values using context, it's important to test the functionality to ensure that the defaults are applied correctly.
Testing Steps:
'Trigger the Action': Use the action that sets the default values.
'Verify Defaults': Ensure that the form fields are pre-populated with the expected default values.
Odoo allows you to combine domains and context to create sophisticated, user-friendly menus and actions. For example, you can create a menu that filters records using a domain and opens a form with pre-set default values using context.
Here’s an example of an action that filters invoices for a specific customer and sets the default values for new records:
<record id="action_invoices_for_customer_1" model="ir.actions.act_window">
<field name="name">Customer 1 Invoices</field>
<field name="res_model">account.move</field>
<field name="view_mode">tree,form</field>
<field name="domain">[('partner_id', '=', 1)]</field>
<field name="context">{'default_partner_id': 1, 'default_move_type': 'out_invoice'}</field>
</record>
Explanation:
Domain: Filters the invoices to only show those related to the customer with ID '1'.
Context: Pre-sets the partner and move type when creating a new invoice.
Link this action to a menu to create a dedicated section for managing invoices for a specific customer:
<menuitem id="menu_customer_1_invoices" name="Customer 1 Invoices" parent="account.menu_finance" action="action_invoices_for_customer_1"/>
Install or upgrade your module and test the combined domain and context functionality:
Navigate to the Menu: Go to the "Customer 1 Invoices" menu.
Verify Filter: Ensure that only invoices for Customer 1 are displayed.
Test Default Values: Click to create a new invoice and confirm that the form is pre-populated with the correct default values.
Now that you've mastered the basics of applying domains to menus and setting default values using context, let's explore some advanced use cases and best practices.
In some scenarios, you may need to create dynamic domains or context values that change based on the user's input or other conditions.
Example: Dynamic Domain Based on User Input
def get_dynamic_domain(self):
user_country_id = self.env.user.partner_id.country_id.id
return [('country_id', '=', user_country_id)]
Example: Dynamic Context Based on User Input
def get_dynamic_context(self):
user = self.env.user
return {'default_country_id': user.partner_id.country_id.id}
You can use computed fields in your domains and context to create more complex conditions. For example, you might filter records based on a computed field that calculates a score or sets a default based on a calculation.
Keep It Simple: Avoid overcomplicating domains and context. Use them judiciously to enhance user experience without confusing users.
Test Thoroughly: Always test your domains and context in various scenarios to ensure they work as expected.
Document Your Code: Clearly document the purpose of each domain and context to make maintenance easier.
Applying domains to menus and setting default values using context in Odoo are essential techniques for creating a streamlined and user-friendly ERP system. By following the steps outlined in this blog, you can customize Odoo to meet specific business requirements, ensuring that users see the most relevant data and have a more efficient experience when entering information.
These powerful tools not only enhance the usability of the system but also allow for sophisticated filtering and data management that can greatly benefit any business using Odoo. Whether you’re an experienced Odoo developer or just getting started, mastering domains and context will enable you to build more tailored and effective solutions.
Your email address will not be published. Required fields are marked *