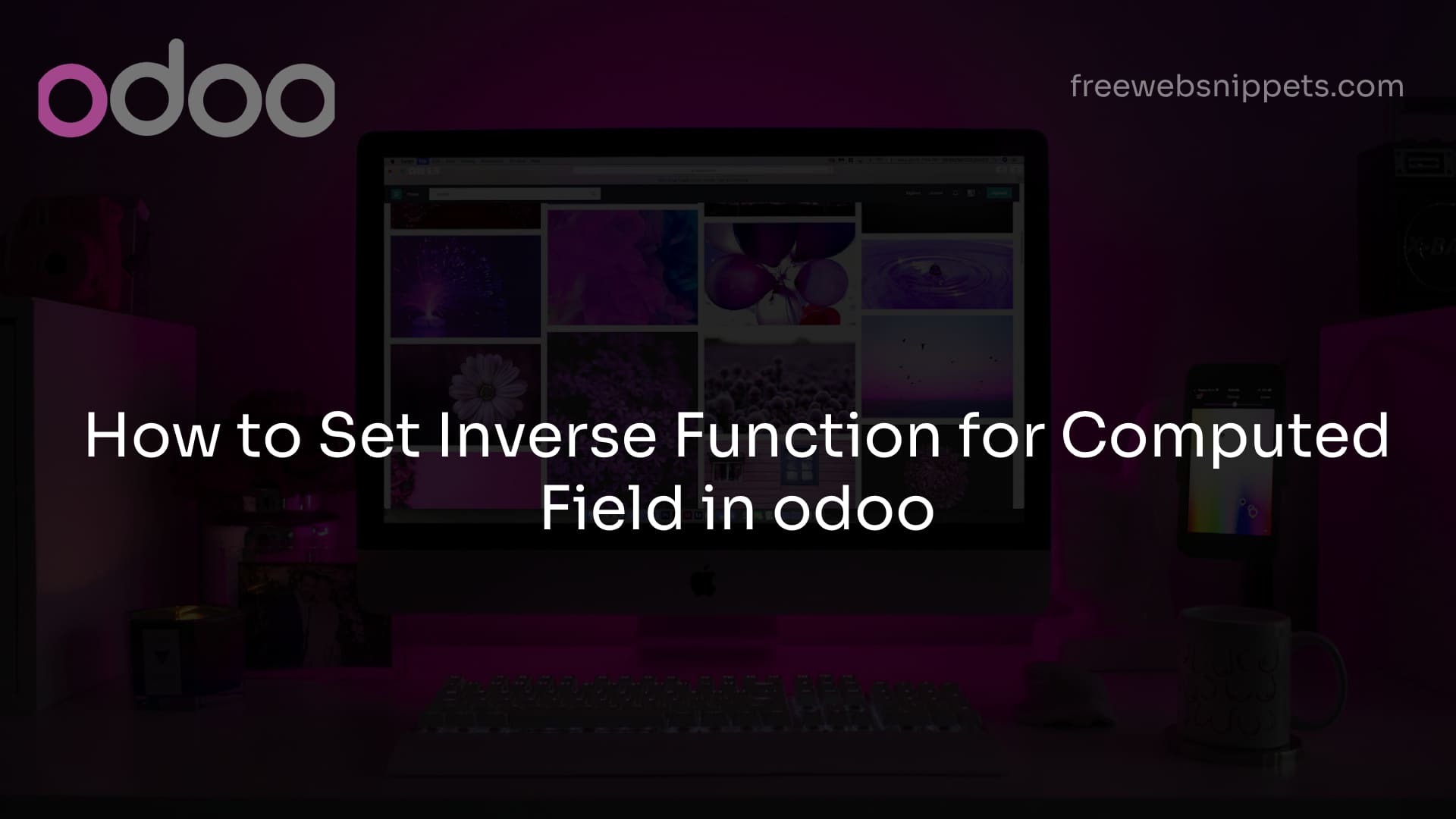
Discover how to create compute fields in Odoo with our comprehensive guide. Enhance your ERP system with dynamic calculations for improved data management.
Odoo, one of the most popular ERP platforms, is known for its flexibility and customization capabilities. A common customization need is to add "compute fields"—dynamic fields that are calculated based on other field values. In this guide, we’ll explore how to create a compute field in Odoo, making your modules more powerful and efficient.
A compute field in Odoo is a field whose value is calculated dynamically by the system rather than being stored directly in the database. The value of this field is computed based on the logic you define.
Let’s walk through creating a compute field in an Odoo model. For this example, we'll calculate the total price of an order line based on quantity and unit price.
The first step is to define the compute field in your model by adding it to your class. A computed field is defined similarly to other fields, but it requires a compute parameter that points to the method responsible for calculating the field value.
Here’s an example of a simple computed field:
from odoo import models, fields, api
class SaleOrderLine(models.Model):
_inherit = 'sale.order.line'
total_price = fields.Float(string="Total Price", compute="_compute_total_price")
@api.depends('product_uom_qty', 'price_unit')
def _compute_total_price(self):
for line in self:
line.total_price = line.product_uom_qty * line.price_unit
The @api.depends decorator is essential when creating compute fields in Odoo. This decorator informs Odoo which fields should trigger the recalculation of the compute field. In our example, the total_price field depends on product_uom_qty and price_unit.
Inside the compute method, you can perform any calculations needed for the compute field. The key is to loop through the records and assign the calculated value to each one. Here's the compute method we used in the example above:
def _compute_total_price(self):
for line in self:
line.total_price = line.product_uom_qty * line.price_unit
This method takes the quantity of the product and multiplies it by the unit price to get the total price.
By default, computed fields in Odoo are not stored in the database. However, if you want the value of the computed field to be stored, you can set the store=True parameter:
total_price = fields.Float(string="Total Price", compute="_compute_total_price", store=True)
Storing computed fields can be useful if you need to search or sort based on that field, but be mindful that it also increases storage requirements.
Once the compute field is defined in the model, you’ll want to display it in your views (forms, lists, etc.). To do this, add the field to the view definition:
<record id="view_order_form" model="ir.ui.view">
<field name="name">sale.order.line.form</field>
<field name="model">sale.order.line</field>
<field name="arch" type="xml">
<form string="Sale Order Line">
<group>
<field name="product_uom_qty"/>
<field name="price_unit"/>
<field name="total_price"/>
</group>
</form>
</field>
</record>
After adding the computed field and updating the view, it's time to test. You can create a new sales order line and see if the total_price field gets automatically updated based on the product quantity and unit price.
Adding compute fields in Odoo is a powerful way to enhance the functionality of your modules. With this step-by-step guide, you should now be able to create your own computed fields and leverage them to optimize your Odoo models. Happy coding!
Your email address will not be published. Required fields are marked *