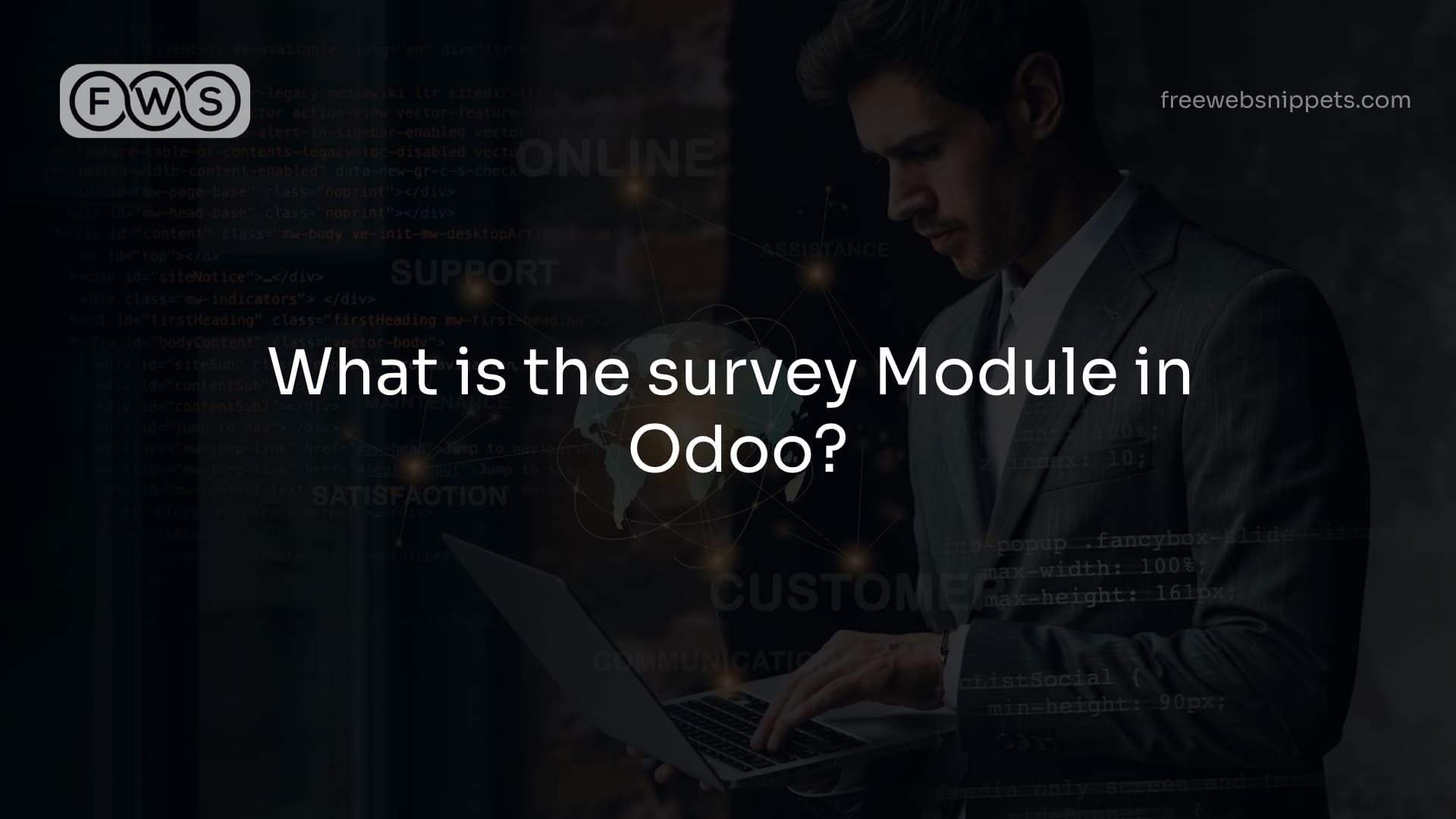
Learn how to integrate Odoo with external apps using the Odoo External API. Discover how to use XML-RPC & JSON-RPC for efficient data management and automation in Odoo.
Odoo's External API lets developers integrate external applications and services. XML-RPC and JSON-RPC protocols are used. Odoo's External API manages data. It also automates workflows and enhances system interoperability. This guide covers using Odoo External API. It also includes how to set up connections and perform operations.
Odoo's External API is a tool. It allows developers to access Odoo models from outside the Odoo environment. Third-party applications can connect with Odoo. The applications can retrieve create update and delete data. This is great for automated integrations. It's also useful for external application syncs.
Connection to Odoo External API requires certain elements:
Example:
import xmlrpc.client
url = 'http://your-odoo-server.com'
db = 'your-database-name'
username = 'api_user'
password = 'your_password'
common = xmlrpc.client.ServerProxy(f'{url}/xmlrpc/2/common')
uid = common.authenticate(db, username, password, {})
Odoo supports both XML-RPC and JSON-RPC protocols. XML-RPC is well-known standard. It is used commonly. On other hand JSON-RPC is more lightweight. It is often favored for web-based applications.
Most common API operations include:
models = xmlrpc.client.ServerProxy(f'{url}/xmlrpc/2/object')
partners = models.execute_kw(db, uid, password, 'res.partner', 'search_read', [[['is_company', '=', True]]], {'fields': ['name', 'country_id'], 'limit': 5})
for partner in partners:
print(partner)
In this script connection is made to res.partner model in Odoo. Companies are searched for and their names and countries are retrieved.
JSON-RPC may be better than XML-RPC for applications that use web. This offers more efficiency. Now consider an example of a search_read operation. This is done with JSON-RPC.
import json
import requests
url = 'http://your-odoo-server.com/jsonrpc'
headers = {'Content-Type': 'application/json'}
data = {
'jsonrpc': '2.0',
'method': 'call',
'params': {
'service': 'object',
'method': 'execute_kw',
'args': [db, uid, password, 'res.partner', 'search_read', [[['is_company', '=', True]]], {'fields': ['name', 'country_id'], 'limit': 5}]
},
'id': 1,
}
response = requests.post(url, headers=headers, data=json.dumps(data))
print(response.json())
A JSON-RPC request is used. The request does a search_read operation. This operation is done a bit differently than how XML-RPC does it. Here, it's done in a more compact format.
By API you can make records in Odoo automatically. For example, creating a new customer record in res.partner model. You can do this with the following command.
partner_id = models.execute_kw(db, uid, password, 'res.partner', 'create', [{'name': "New Customer", 'is_company': True}])
print("Created partner ID:", partner_id)
It creates a new company record. The record is in res.partner model. The company's name is set as “New Customer”.
Updating and deleting records is simple. Updating is also known as writing. Deletion is termed as unlinking. Let's see how it is done.
models.execute_kw(db, uid, password, 'res.partner', 'write', [[partner_id], {'name': "Updated Customer Name"}])
This updates the partner’s name. It then removes the record.
models.execute_kw(db, uid, password, 'res.partner', 'unlink', [[partner_id]])
Removed the record. This deletes the record entirely.
When using Odoo External API, proper error handling is key. This is especially true for network-related errors and permission issues. A few key points to remember:
try:
partner_id = models.execute_kw(db, uid, password, 'res.partner', 'create', [{'name': "Safe Customer"}])
except xmlrpc.client.Fault as e:
print("Error:", e)
Odoo External API allows powerful ways to integrate. It can extend Odoo functionalities with other applications. This is achieved particularly through use of XML-RPC or JSON-RPC protocols. These protocols help with management of data within Odoo’s models from outside system.
Understanding how to connect is crucial. You also need to know how to read create and update records. Knowing how to delete records is equally essential. By mastering these actions you can create robust and flexible integrations for your Odoo environment. This gives you necessary skills to enhance and modify the Odoo system according to your requirements.
Your email address will not be published. Required fields are marked *